Ask Sage API
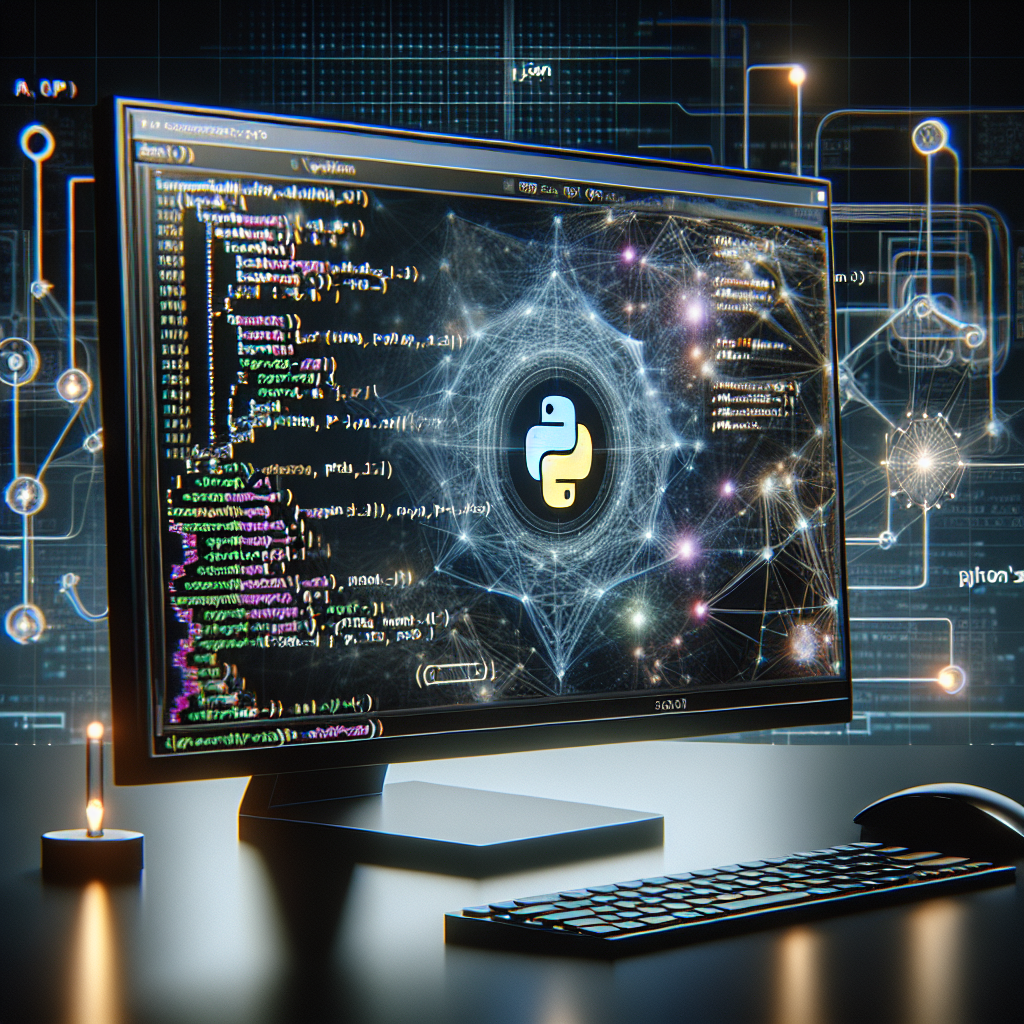
The Ask Sage Python Client is a Python library that provides an easy-to-use methods for interacting with the Ask Sage API. The Python client allows you to query models, and perform other operations using Python code.
Table of contents
API Python Client Documentation
The documentation for the Ask Sage Pythonn Client can be found Here:
- Python API Client: https://pypi.org/project/asksageclient/
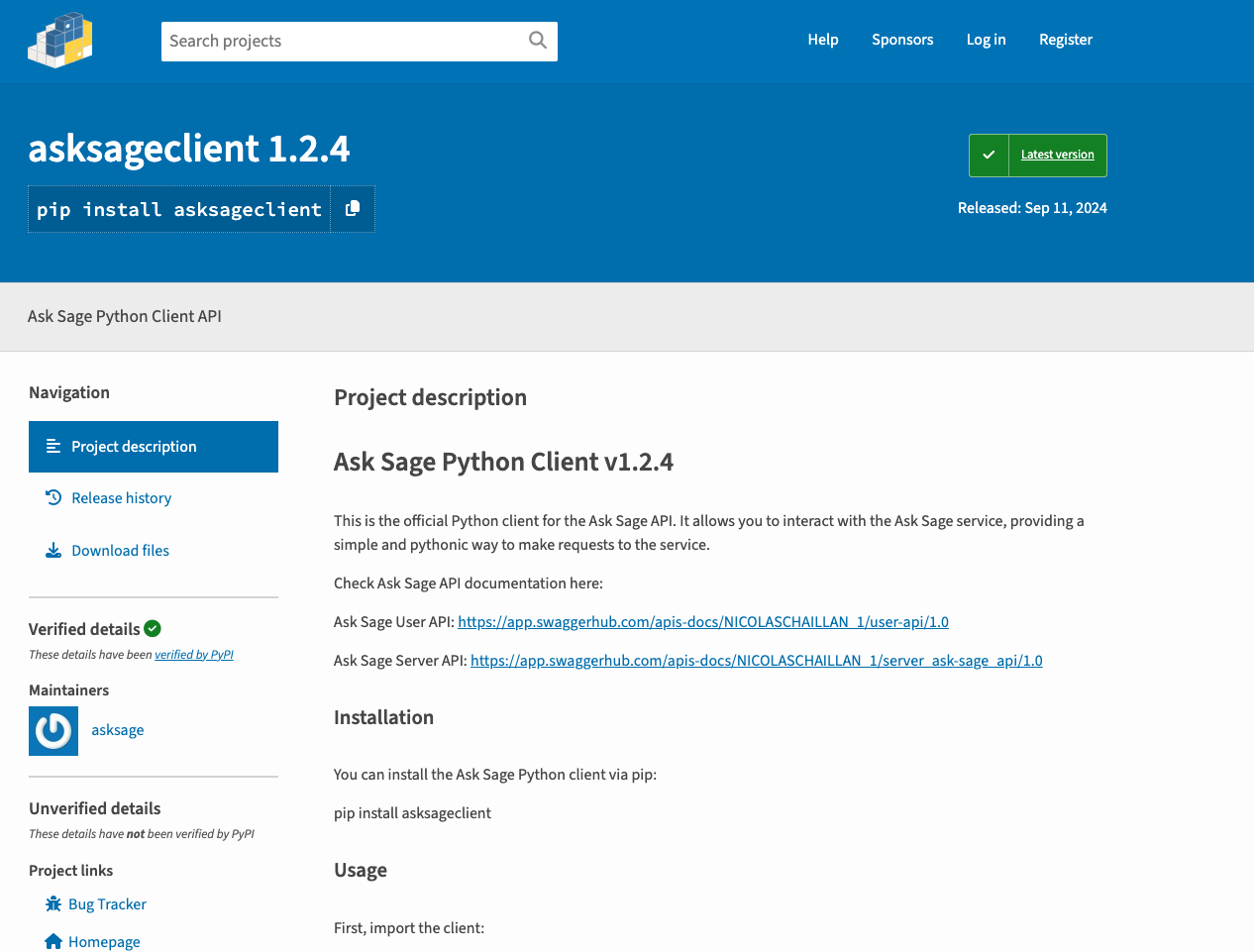
Python Client Endpoints
Function Name | Description |
---|---|
get_models | Get the available models from the Ask Sage service. |
add_dataset | Adds a new dataset |
delete_dataset | Deletes a specified dataset |
assign_dataset | Assigns a dataset |
get_user_logs | Retrieves all logs for user |
get_user_logins | Retrieves login information for a specific user |
query | Interact with the /query endpoint of the Ask Sage API. |
query_with_file | Executes a query using a file |
query_plugin | Executes a query using a specific plugin |
execute_plugin | Executes a plugin with the provided content |
follow_up_questions | Interact with the /follow-up-questions endpoint of the Ask Sage API. |
tokenizer | Interact with the /tokenizer endpoint of the Ask Sage API. |
get_personas | Get the available personas from the Ask Sage service. |
get_datasets | Get the available datasets from the Ask Sage service. |
get_plugins | Get the available plugins from the Ask Sage service. |
count_monthly_tokens | Get the count of monthly training tokens spent for this user from the Ask Sage service. |
count_monthly_teach_tokens | Counts the number of teach tokens used in a month |
train | Train the model based on the provided content. |
train_with_file | Train the dataset based on the provided file. |
file | Upload a file to the Ask Sage service. |
Setup and Installation
The API key and email can be used to authenticate the user and grant access via the Ask Sage Python Client. The API key and user email will be included in the request headers.
For example, the following code snippets demonstrates how to create an instance of the Ask Sage Client class and authenticate with the Ask Sage API using the API key and email:
Step 1: Create a credentials file in JSON format with the API key and user email:
{
"credentials": {
"api_key": "YOUR_API_KEY", // The API key for the Ask Sage API, which can be obtained from the Ask Sage website
"Ask_sage_user_info": {
"username": "YOUR_EMAIL" // The email address of the user
}
}
}
Step 2: Load the credentials from the JSON file and create an instance of the Ask SageClient class:
import json # Import the json module to work with JSON data
import requests # Import the requests library to send HTTP requests
from asksageclient import AskSageClient # Import the AskSageClient class from the asksageclient module
# Function to load credentials from a JSON file
def load_credentials(filename):
try:
with open(filename) as file:
return json.load(file)
except FileNotFoundError:
raise FileNotFoundError("The credentials file was not found.")
except json.JSONDecodeError:
raise ValueError("Failed to decode JSON from the credentials file.")
# Load the credentials
credentials = load_credentials('../../credentials.json')
# Extract the API key, and email from the credentials to be used in the API request
api_key = credentials['credentials']['api_key']
email = credentials['credentials']['Ask_sage_user_info']['username']
"""
class AskSageClient(
email: email, # The email address of the user
api_key: api_key, # The API key for the Ask Sage API, which can be obtained from the Ask Sage website
user_base_url: str = 'https://api.asksage.ai/user', # The base URL for the user API
server_base_url: str = 'https://api.asksage.ai/server' # The base URL for the server API
)
"""
ask_sage_client = AskSageClient(email, api_key) # Create an instance of the Ask Sage Client class with the email and api_key
Following this setup the user can now interact with the Ask Sage API using the Ask Sage Client object.