API Endpoints
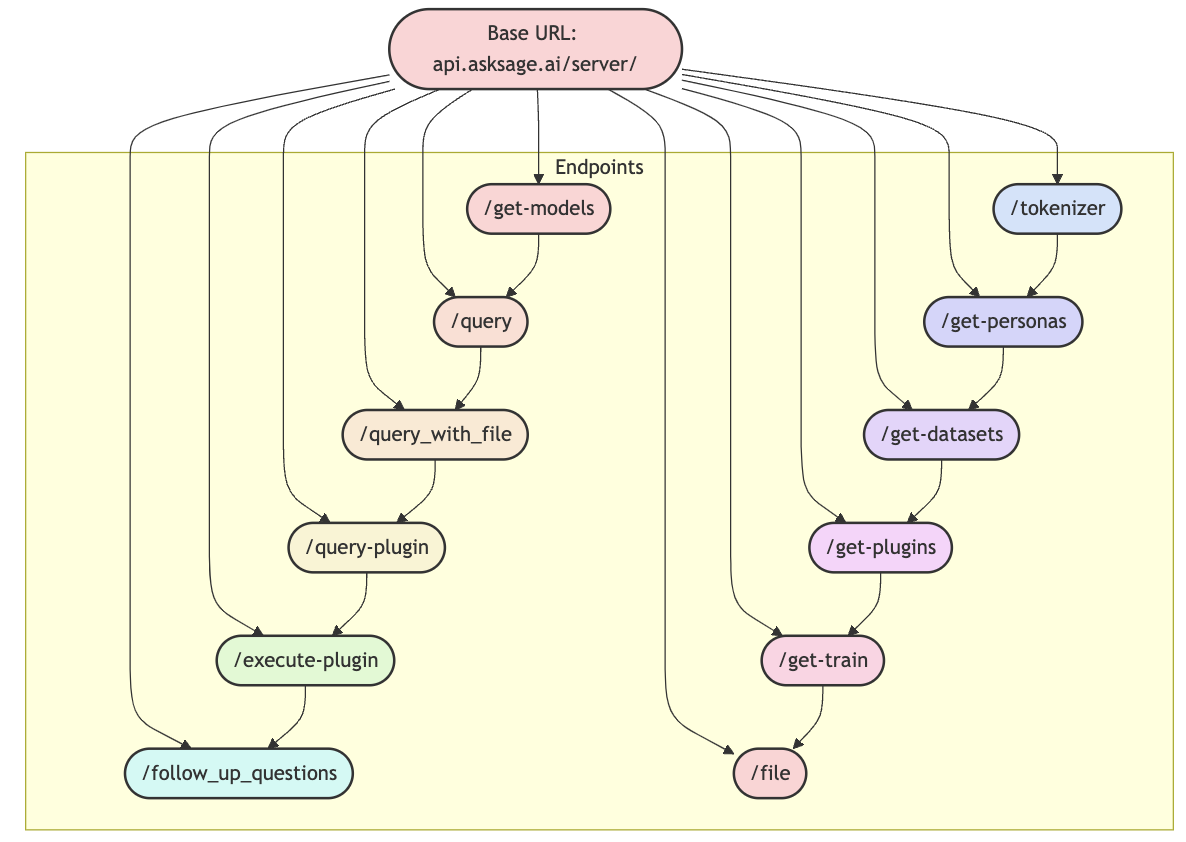
In this section will cover how to access the Ask Sage API and the available endpoints.
Table of contents
API Endpoints
The Ask Sage API is a RESTful API that provides access to the Ask Sage platform. The API is divided into two main parts: the User API and the Server API. Each API has its own set of endpoints and functionalities.
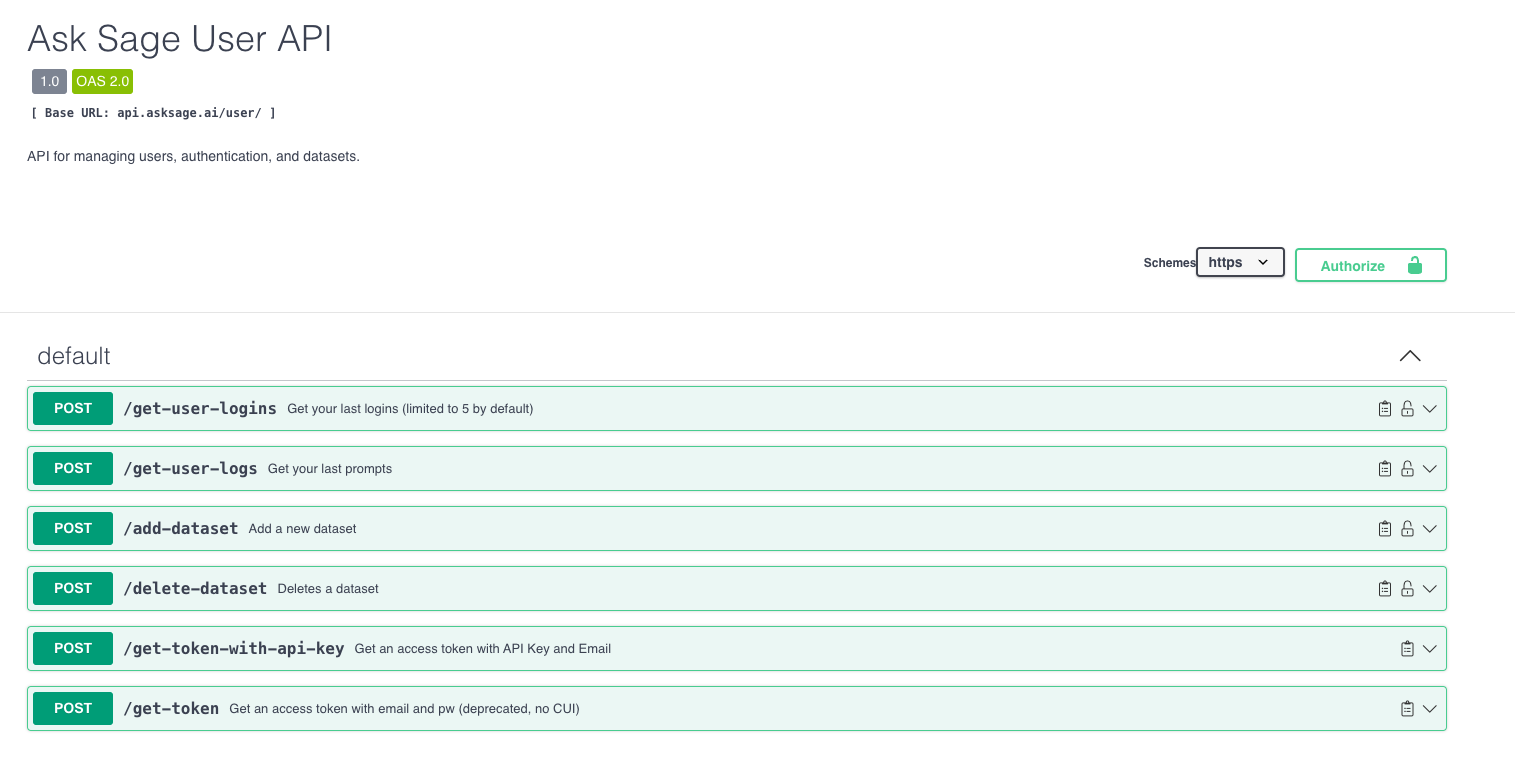
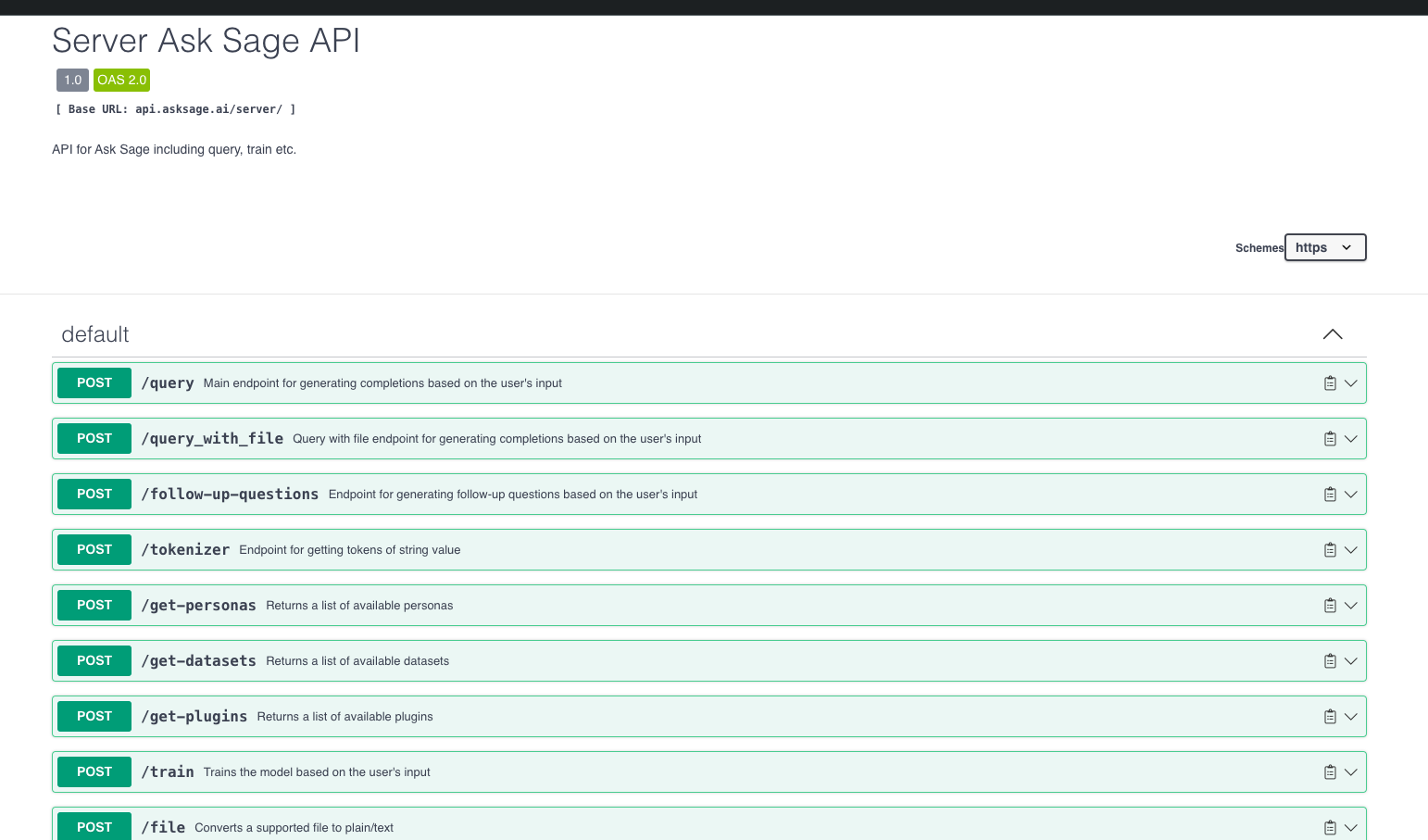
User API Endpoints:
The User API is used for managing users, authentication, and datasets. We have the following Swagger documentation for the User API:
- User - Swagger Documentation
- The Base URL for the User API is: [ Base URL: api.asksage.ai/user/ ]
Make sure to use the correct Base URL associated with your tenant.
The following table shows the available endpoints in the User API:
Endpoint | Description |
---|---|
/get-token-with-api-key | Get an access token with API Key and email |
/get-user-logins | Get your last logins (limited to 5 by default) |
/get-user-logs | Get your last prompts |
/add-dataset | Add a new dataset |
/delete-datasets | Deletes a dataset |
Server API Endpoints:
The Server API is used for managing the server, including the Ask Sage API. We have the following Swagger documentation for the Server API:
- Server - Swagger Documentation
- The Base URL for the Server API is: [ Base URL: api.asksage.ai/server/ ]
Make sure to use the correct Base URL associated with your tenant.
The following table shows the available endpoints in the Server API:
Endpoint | Description |
---|---|
/get-models | Returns a list of available models via the Ask Sage services |
/query | Main endpoint for generating completions based on the user’s input |
/query_with_file | Query with file endpoint for generating completions based on the user’s input |
/query-plugin | Query with plugin endpoint for generating completions based on the user’s input |
/execute-plugin | Execute a plugin with the provided content |
/follow_up_questions | Endpoint for generating follow-up questions based on the user’s input |
/tokenizer | Endpoint for getting tokens of string value |
/get-personas | Endpoint for getting the tokens of string |
/get-datasets | Returns a list of available datasets |
/get-plugins | Returns a list of available plugins |
/train | Trains the model based on the user’s input |
/file | Converts a supported file to plain/text |
The Swagger documentation provides a setup interface where you can test the API endpoints and see the responses.
Base URLs may change, depending on the environment you are working on. If you need assistance, please reach out to us at support@asksage.ai.
API Authentication Set Up
There are two methods to which a user can authenticate with the Ask Sage Endpoints:
- 24 Hour - Access Token: This method generates an access token that is valid for 24 hours. The access token is used to authenticate requests to the Ask Sage API.
- Static API Key: This method uses a static API key to authenticate requests to the Ask Sage API. The API key is passed in the header where the token is expected.
Both methods are shown below:
1. 24 Hour - Access Token
An access token is generated using the API key
and email address
with the endpoint ‘/get-token-with-api-key’. The access token is valid for 24 hours and can be used to authenticate requests to the Ask Sage API.
This is a more secure method of authentication as the access token is only valid for a limited time and must be regenerated periodically.
Here is a sample code snippet in Python that demonstrates how to obtain an access token using your email address and API key:
import requests
# Define the endpoint URL
url = "https://api.asksage.ai/user/get-token-with-api-key"
# Define the payload with the user's email and API key
payload = {
"email": "your_email@your_domain.com",
"api_key": "sdfsdfsfr23456789" # Your API key generated from the Ask Sage platform
}
# Set the headers, if required (e.g., Content-Type)
headers = {
"Content-Type": "application/json"
}
# Make the POST request
response = requests.post(url, json=payload, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON response
data = response.json()
# raw response
print(data)
# Extract the access token only from the response
access_token = data['response']['access_token']
print(access_token)
The generated access token can be used to authenticate requests to the Ask Sage API for the next 24 hours. Here is an example of how to use the access token to authenticate a request to any of the Ask Sage API endpoints:
import requests
# Define the access token obtained from the previous request - Note: You would want to store this securely and not hardcode it in your script/file.
access_token = "fghjkl4567890" # Replace with the actual access token
# Define the endpoint URL
url = "https://api.asksage.ai/user/get-user-logins" # Replace with the actual base URL of the API
# Define the payload with the limit parameter
payload = {
"limit": 1 # Replace with the desired limit (max is 100)
}
# Set the headers, including the Authorization header with the Bearer token
headers = {
"x-access-tokens": access_token,
"Content-Type": "application/json"
}
# Make the POST request
response = requests.post(url, json=payload, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON response
data = response.json()
print("User Logins:", data)
else:
print(f"Failed to get user logins. Status code: {response.status_code}")
print(f"Response: {response.text}")
2. Static API Key
Instead of generating an access token every time you need to access the API, you can pass the static API key
where the token is expected (This is the API Key
generated from the Ask Sage platform). This is not as secure as generating a 24 hour access token, but it is a valid option for some use cases.
Note: The expected header variable is
x-access-tokens
and the value is theAPI key
. There is no time limit on theAPI key
, but it is recommended to regenerate it periodically for security reasons.
import requests
# Define the access token obtained from the previous request - Note: You would want to store this securely and not hardcode it in your script/file.
access_token = api_key # Replace with the actual API key generated from the Ask Sage platform
# Define the endpoint URL
url = "https://api.asksage.ai/user/get-user-logins" # Replace with the actual base URL of the API
# Define the payload with the limit parameter
payload = {
"limit": 1 # Replace with the desired limit (max is 100)
}
# Set the headers, including the Authorization header with the Bearer token
headers = {
"x-access-tokens": access_token,
"Content-Type": "application/json"
}
# Make the POST request
response = requests.post(url, json=payload, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON response
data = response.json()
print("User Logins:", data)
else:
print(f"Failed to get user logins. Status code: {response.status_code}")
print(f"Response: {response.text}")